How to Write Good Code
Dev
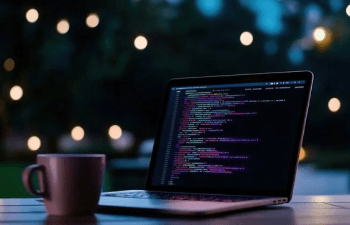
Writing good code is about more than just making it work; it's about creating code that’s efficient, readable, maintainable, and adaptable to future requirements. Good code not only makes your software more resilient but also benefits your team by making it easier to understand and extend. This guide will cover best practices and techniques to help you write high-quality code, from planning to testing and beyond.
1. Planning Your Code
Good code starts with good planning. Before diving into writing code, take time to understand the requirements, design an outline, and think through the structure.
Break Down the Problem
Identify the main goals and the smaller tasks required to achieve them. Breaking down problems helps you avoid unnecessary complexity, which leads to better code clarity.
Choose the Right Data Structures
Data structures directly affect your code’s performance and readability. Carefully select the ones that match your requirements. For example, use arrays for ordered collections, hash tables for fast data retrieval, and stacks or queues when order matters.
Define a Clear Architecture
Decide on an architecture that’s suitable for the project scale. Consider using modular structures like MVC (Model-View-Controller) or MVP (Model-View-Presenter) for larger applications, as they separate concerns and make the code easier to manage and maintain.
2. Writing Readable Code
Readable code is a hallmark of good code. It should be understandable by other developers (and your future self) without extensive explanations.
Use Descriptive Names
Choose variable, function, and class names that clearly indicate their purpose. Avoid vague names like `x`
or `temp`
—opt for names that describe the data they represent, such as `userEmail`
or `orderTotal`
.
Avoid Magic Numbers and Hardcoded Values
Use constants to store values instead of hardcoding numbers or strings directly in the code. This makes your code easier to understand and update. For example, instead of `if (score > 90)`
, use `const PASSING_SCORE = 90; if (score > PASSING_SCORE)`
.
Write Concise and Focused Functions
Functions should do one thing and do it well. Avoid lengthy functions by breaking down tasks into smaller functions. This makes your code more modular and promotes reusability. A common guideline is the Single Responsibility Principle, which states that each function or module should have one responsibility.
Comment Wisely
Use comments to explain why you’re doing something rather than what you’re doing. Avoid redundant comments that restate what the code is already doing. For example:
// Bad comment
int x = 0; // Set x to zero
// Good comment
int x = 0; // Initialize counter for user actions
3. Adopting Coding Standards and Style Guides
Following a coding style guide helps maintain a consistent look throughout the codebase. Consistency makes it easier to read, navigate, and spot errors.
Use a Style Guide
A style guide defines conventions such as indentation, naming conventions, and spacing. Many languages have popular style guides like Google JavaScript Style Guide or PEP 8 for Python. Choose one and stick to it, as this uniformity enhances readability.
Leverage Linters and Formatters
Linters and formatters like ESLint for JavaScript and Prettier for general formatting can enforce code style rules automatically. They catch common errors and ensure that code is well-organised. Automating style checks with linters reduces manual corrections and promotes clean code.
4. Writing Efficient Code
Efficiency in code means writing logic that performs optimally without using unnecessary resources. Efficient code reduces load times, optimizes memory usage, and makes applications responsive.
Optimize Loops and Conditionals
Minimize nested loops and conditionals where possible. Nested loops can lead to O(n²) complexity, which slows down performance. Try using alternative approaches like hash maps for searching, or break down complex conditions into simpler, more manageable statements.
Avoid Redundant Code
Don’t Repeat Yourself (DRY) is a principle that emphasizes reusability by reducing redundancy. For example, if the same piece of code appears in multiple places, create a function or module that you can call instead. This not only reduces the codebase but also makes it easier to maintain.
Manage Memory Wisely
Memory management is particularly important for languages like C++ that don’t have automatic garbage collection. Freeing unused memory, avoiding memory leaks, and using memory-efficient data structures can improve both performance and stability.
5. Embracing Modularization and Reusability
Modular code is organized into self-contained units, like classes, functions, or modules. This structure makes it easier to understand and reuse code across different parts of an application.
Use Modules for Code Organization
Modules divide code into logical sections based on functionality. For example, have a separate module for handling data, another for UI elements, and another for API calls. This promotes code reuse and keeps components organised.
Follow the Single Responsibility Principle (SRP)
The SRP is a key concept in modular design. Each function, class, or module should have a single responsibility. This reduces interdependency and makes your code more adaptable to changes.
Implement Reusable Components
For front-end development, reusable components in frameworks like React or Vue.js make it easy to create consistent UIs. Instead of hardcoding elements repeatedly, create a single component and reuse it wherever needed.
6. Ensuring Robust Testing
Testing is an integral part of writing good code. It ensures that your code works as expected and prevents issues before they reach production.
Write Unit Tests
Unit tests verify individual components or functions. They’re crucial for catching bugs early and ensuring that each part of your code behaves as expected. Tools like Jest for JavaScript or JUnit for Java help automate testing and provide a structure for unit testing.
Embrace Test-Driven Development (TDD)
In Test-Driven Development, you write tests before writing the actual code. This approach ensures that your code is driven by requirements and forces you to think about edge cases and errors. TDD also creates a robust suite of tests that make refactoring safer.
Perform Code Reviews
Code reviews are a valuable way to catch mistakes, share knowledge, and enforce code standards. When code is reviewed by peers, errors or inefficiencies that a single developer might miss become more visible. Regular code reviews help maintain code quality across the team.
7. Prioritizing Security and Error Handling
Security and error handling are vital for stable applications, especially for web development, where vulnerabilities can lead to serious breaches.
Implement Secure Coding Practices
Avoid exposing sensitive data, sanitize user input, and implement proper authentication. For example, avoid directly including sensitive information in your code or using weak hashing algorithms.
Use Error Handling Techniques
Handling errors prevents unexpected crashes and improves user experience. Use try-catch blocks to handle exceptions gracefully. For API or database interactions, ensure your code can handle unexpected responses, timeouts, or errors effectively.
try {
// Attempt code that may throw an error
} catch (error) {
console.error("An error occurred:", error);
}
Log Errors Effectively
Effective logging helps track errors and their root causes. In a production environment, make sure to log errors without exposing sensitive information. For complex applications, logging frameworks can manage log files and send alerts for critical issues.
8. Refactoring Regularly
Refactoring improves the code’s structure without changing its behavior. It makes code easier to understand and adapt to new requirements.
Simplify Complex Code
Refactoring allows you to simplify complex code by breaking it down into smaller, easier-to-read functions. This makes the codebase more manageable and easier for others to understand.
Remove Redundant Code
If certain parts of the codebase are no longer needed or used, remove them. This reduces clutter and improves performance. Also, refactor code to reuse functions instead of writing redundant code blocks.
Perform Regular Maintenance
Make refactoring a regular part of your development process. Avoid leaving technical debt, which is the accumulation of “quick fixes” that later require revisiting. Routine maintenance ensures your code remains scalable and manageable.
9. Documenting Your Code
Documentation is essential for good code. Well-documented code is easier to understand, onboard, and maintain.
Write Clear Documentation for Functions and Classes
Include a brief explanation at the start of each function, class, or module. Documentation should cover what the function does, the input parameters, and the expected output.
Create a Project-Level README
A README file provides an overview of the project, installation instructions, and a quick-start guide. Include relevant information like dependencies, setup instructions, and how to use the software.
Conclusion
Writing good code isn’t just about getting it to work; it’s about writing it in a way that makes it easy to understand, maintain, and extend. By following these best practices, from planning and writing readable code to prioritizing security and refactoring regularly, you’ll be creating code that stands the test of time. Not only will it benefit you, but it will also help your team and contribute to the overall quality of your projects. With a commitment to writing clean, efficient, and well-documented code, you’re well on your way to becoming a better developer.